The CodeSite Logging System gives developers deeper insight into how their code is executing, enabling them to locate problems more quickly and monitor how well their code is performing. Developers instrument their code using CodeSite loggers which send CodeSite messages to a live display or log file during program execution. All kinds of information can be encoded in a CodeSite message, and the CodeSite Live Viewer and File Viewer are specially designed tools for analyzing CodeSite messages. CodeSite is not only effective during development and testing; in production environments, CodeSite provides valuable information to support staff and developers.
Note: EurekaLog itself can stream to CodeSite-compatible format, though these features are very limited. We don't recommend to use EurekaLog's logging capabilities when you have access to a proper logging framework.
CodeSite to EurekaLog
If you are using CodeSite in your application - it may be useful to get CodeSite output as part of your EurekaLog's crash reports, so you will get a better understanding of execution flow of your application before crash. You can attach the log file from CodeSite with the following code example:
Important Note: example below will add a new file with log output from CodeSite. The new file will be added inside EurekaLog's bug report that is being send to developers. In other words, you have to set up sending to receive this file. The local EurekaLog's report do not store any additional files. If you wish to capture .elp file locally for testing purposes - see this example.
uses
CodeSiteLogging, // for CodeSite classes and routines
EException, // for TEurekaExceptionInfo
ESysInfo, // for GetFolderTemp
EEvents; // for RegisterEventZippedFilesRequest
var
// File name for CodeSite log file
GCodeSiteFileName: String;
// Initialize CodeSite to save log to file
// This is just an example
// You may replace/customize it
// Please, refer to CodeSite documentation
procedure InitCodeSite;
var
Dest: TCodeSiteDestination;
begin
GCodeSiteFileName := GetFolderTemp +
ChangeFileExt(ExtractFileName(ParamStr(0)), '.csl');
Dest := TCodeSiteDestination.Create(CodeSite);
Dest.LogFile.FilePath := ExtractFilePath(GCodeSiteFileName);
Dest.LogFile.FileName := ExtractFileName(GCodeSiteFileName);
Dest.LogFile.Active := True;
CodeSite.Destination := Dest;
end;
// Will be called when EurekaLog wants to
// add additional files to packed bug report file (.elp)
procedure PackLogFile(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
const ATempFolder: String;
AAttachedFiles: TStrings;
var ACallNextHandler: Boolean);
var
LFileName: String;
begin
// Get a temporary filename
LFileName := ATempFolder + ExtractFileName(GCodeSiteFileName);
// Copy the log file
CopyFile(PChar(GCodeSiteFileName), PChar(LFileName), False);
// Pack the file to EurekaLog's crash report
AAttachedFiles.Add(LFileName);
end;
initialization
// Initialize CodeSite to save log to file
InitCodeSite;
// Ask EurekaLog to add more files to .elp reports
RegisterEventZippedFilesRequest(nil, PackLogFile);
end.
When you receive crash report from EurekaLog - the CodeSite log will be shown as file attach:
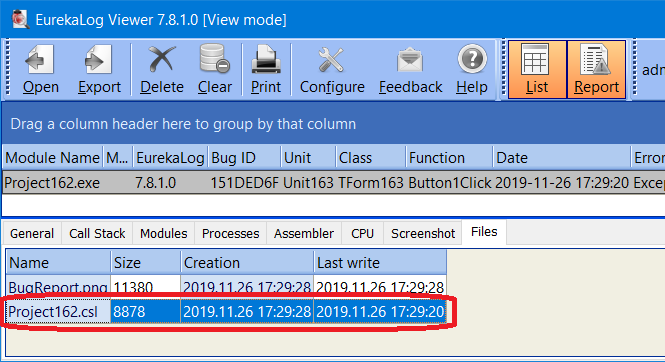
CodeSite log file inside EurekaLog's crash report
Double-click log file to view in CodeSite Viewer tool
EurekaLog to CodeSite
Alternatively, you may want to set up a reverse integration. E.g. you may want to have EurekaLog's crash information inside your CodeSite log files. Use example below:
uses
CodeSiteLogging, // for CodeSite classes and routines
EException, // for TEurekaExceptionInfo
EEvents; // for RegisterEventExceptionNotify
// Tell EurekaLog to log crash info with CodeSite
procedure LogExceptionToCodeSite(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
var AHandle: Boolean;
var ACallNextHandler: Boolean);
var
CallStack: TStringList;
begin
// Check if exception is of Exception class (usually: yes)
if AExceptionInfo.ExceptionNative and
(AExceptionInfo.ExceptionObject <> nil) and
TObject(AExceptionInfo.ExceptionObject).InheritsFrom(Exception) then
// IMPORTANT NOTE: Please note that the .ExceptionObject may be unavailable even for Delphi exceptions!
// For example, if the exception object was already deleted:
//
// try
// raise Exception.Create('Inner Exception'); // - will be deleted
// except
// on E: Exception do
// raise Exception.Create('Outer Exception');
// end;
//
// See also.
// That is why we check for NIL in the example above.
// For this reason we highly recommend to use properties of AExceptionInfo when possible,
// Such as .ExceptionClass and .ExceptionMessage
CodeSite.SendException(
AExceptionInfo.ExceptionClass,
Exception(AExceptionInfo.ExceptionObject))
else
// Log other "strange" exceptions
CodeSite.SendError('[%s] %s',
[AExceptionInfo.ExceptionClass,
AExceptionInfo.ExceptionMessage]);
// Log exception's call stack
CallStack := TStringList.Create;
try
CallStack.Assign(AExceptionInfo.CallStack);
CodeSite.Send('Exception Call Stack', CallStack);
finally
FreeAndNil(CallStack);
end;
// You may also log other properties of AExceptionInfo
// Or you can use routines from ESysInfo to log process and environment info
// Of you can use BuildBugReport function to compose bug report text
end;
initialization
// Tell EurekaLog to log crash info with CodeSite
RegisterEventExceptionNotify(nil, LogExceptionToCodeSite);
end.
See also:
Send feedback...
|
Build date: 2025-05-08
Last edited: 2025-04-01
|
PRIVACY STATEMENT
The documentation team uses the feedback submitted to improve the EurekaLog documentation.
We do not use your e-mail address for any other purpose.
We will remove your e-mail address from our system after the issue you are reporting has been resolved.
While we are working to resolve this issue, we may send you an e-mail message to request more information about your feedback.
After the issues have been addressed, we may send you an email message to let you know that your feedback has been addressed.
Permanent link to this article: https://www.eurekalog.com/help/eurekalog/logging_codesite.php
|
|