You can add simple text information or even arbitrary file(s). Text information will be included right inside .el bug report file, and can be viewed directly in Viewer ("General" tab when viewing report). Files can be added inside packed .elp bug report file, and can be opened by Viewer by double-clicking on file ("Files" tab when viewing report).
Custom file(s)
Important: custom files can only be included in the bug report for sending (.elp files). Local bug reports are simple text files, which can not contain other files.
Register OnZippedFilesRequest event handler:
uses
EEvents;
procedure AttachAdditionalFiles(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
const ATempFolder: String;
AAttachedFiles: TStrings;
var ACallNextHandler: Boolean);
var
FS: TFileStream;
FileName: String;
begin
// Option 1: attach existing file directly:
AAttachedFiles.Add('C:\Windows\win.ini');
// Option 2: create a new file:
FileName := ATempFolder + 'MyCustomFile.bin';
// All files in ATempFolder will be deleted after send
AAttachedFiles.Add(FileName);
FS := TFileStream.Create(FileName, fmCreate or fmShareDenyWrite);
try
FS.WriteBuffer({...});
finally
FreeAndNil(FS);
end;
end;
initialization
RegisterEventZippedFilesRequest(nil, AttachAdditionalFiles);
end.
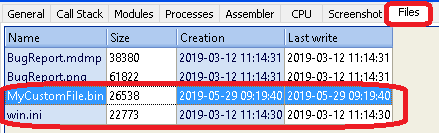
Custom files inside bug report file
Double-click on file to open it in default application.
Custom text info
You can use AddCustomDataToBugReport function to add static data:
procedure TForm1.FormCreate(Sender: TObject);
begin
// ...
AddCustomDataToBugReport('Serial Number', FSerialNumber);
// ...
end;
initialization
AddCustomDataToBugReport('Admin Mode', FindCmdLineSwitch('admin'));
end.
If your data is dynamic (in other words: can change during run-time or depends on the exception, etc.) - register OnCustomDataRequest event handler:
uses
EEvents;
procedure AddMyData(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
ALogBuilder: TObject;
ADataFields: TStrings;
var ACallNextHandler: Boolean);
begin
// Add your own information
// Code below is just an example
ADataFields.Values['Serial'] := ReadYourSerialNumberFromRegistry;
ADataFields.Values['Used Database'] := 'none';
ADataFields.Values['Logged on User'] :=
AExceptionInfo.ExpandEnvVars('%UserName%');
// ...
end;
initialization
RegisterEventCustomDataRequest(nil, AddMyData);
end.
or:
uses
ELogBuilder;
procedure AddMyData(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
ALogBuilder: TBaseLogBuilder;
ADataFields: TStrings;
var ACallNextHandler: Boolean);
begin
// Add your own information
// Code below is just an example
ADataFields.Values['Serial'] := ReadYourSerialNumberFromRegistry;
ADataFields.Values['Used Database'] := 'none';
ADataFields.Values['Logged on User'] :=
AExceptionInfo.ExpandEnvVars('%UserName%');
// ...
end;
initialization
RegisterEventCustomDataRequest(nil, AddMyData);
end.
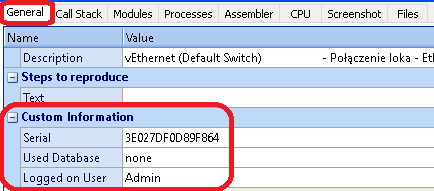
Custom text information in bug report
Note: you have to enable custom information for the bug report. It is already enabled by default, but you should double-check it just in case:
1. | Go to Project / EurekaLog options; |
2. | Go to Bug Report / Content tab; |
3. | Scroll down to the end and enable "Custom information": |
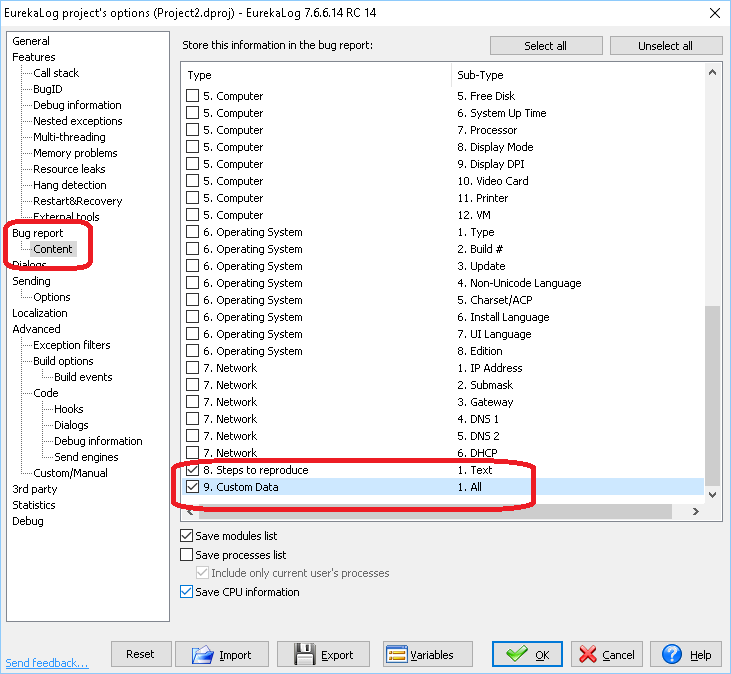
Enabling custom information for bug report
Adding user input from custom dialog
If you want to add custom data to bug report file, and this data is entered by an user in your own custom exception dialog - then you can simply insert/update custom fields in your dialog after showing, and refresh the report. For example:
uses
EException, // for TEurekaExceptionInfo
EDialog, // for TBaseDialog and RegisterDialogClass
EModules, // for CurrentEurekaLogOptions
EEvents, // for RegisterEventCustomDataRequest
ETypes; // for TResponse
type
// Your exception dialog
TMyExeptionDialog = class(TBaseDialog)
protected
function ShowModalInternal: TResponse; override;
public
class function ThreadSafe: Boolean; override;
end;
{ TMyExeptionDialog }
class function TMyExeptionDialog.ThreadSafe: Boolean;
begin
Result := False;
end;
function TMyExeptionDialog.ShowModalInternal: TResponse;
var
S: String;
begin
// Default result
Finalize(Result);
FillChar(Result, SizeOf(Result), 0);
Result.SendResult := srSent;
// Only as example, replace with your actual dialog / form
S := '';
if InputQuery('Exception', 'Enter your data:', S) then
begin
// Fill data for AddUserData below
// This code will associate your data with exception data
// '_My_Field1' - is an arbitrary string
// Using '_My_' prefix is a good idea to avoid collisions
ExceptionInfo.Options.CustomField['_My_Field1'] := S;
// Some other values...
ExceptionInfo.Options.CustomField['_My_Field2'] := S;
ExceptionInfo.Options.CustomField['_My_Field3'] := S;
// Update custom fields
AddCustomData;
// Refresh custom fields in the report
RefreshReport;
end;
end;
// Event handler for OnCustomDataRequest event
procedure AddUserData(const ACustom: Pointer;
AExceptionInfo: TEurekaExceptionInfo;
ALogBuilder: TObject;
ADataFields: TStrings;
var ACallNextHandler: Boolean);
begin
// 'Field1' is an arbitrary name to add to bug report
// '_My_Field1' is the name from your dialog's code above
ADataFields.Values['Field1'] :=
AExceptionInfo.Options.CustomField['_My_Field1'];
ADataFields.Values['Field2'] :=
AExceptionInfo.Options.CustomField['_My_Field2'];
ADataFields.Values['Field3'] :=
AExceptionInfo.Options.CustomField['_My_Field3'];
end;
initialization
// Register your dialog, so it can be used by EurekaLog
RegisterDialogClass(TMyExeptionDialog);
// Switch to your dialog
CurrentEurekaLogOptions.ExceptionDialogType :=
TMyExeptionDialog.ClassName;
// Instruct EurekaLog to add custom fields to bug report
RegisterEventCustomDataRequest(nil, AddUserData);
end.
TBaseDialog.AddCustomData is for adding custom fields to bug report file. This method is placed in dialog class, because dialog class is responsible for the whole exception processing (think about Null dialog): saving bug report, showing visual window, sending report, restarting app, etc. AddCustomData is called before ShowModal. In other words, EurekaLog saves bug report file first, and only then shows visual dialog. That is because if exception dialog fails for any reason - you will still have your bug report (without custom fields though). TBaseDialog.RefreshReport saves bug report again. If report was pre-saved - it will be overwritten. This will update all changes that were made to bug report in memory: custom fields, steps to reproduce, user's e-mail, send status, etc.
See also:
Send feedback...
|
Build date: 2025-05-08
Last edited: 2025-04-01
|
PRIVACY STATEMENT
The documentation team uses the feedback submitted to improve the EurekaLog documentation.
We do not use your e-mail address for any other purpose.
We will remove your e-mail address from our system after the issue you are reporting has been resolved.
While we are working to resolve this issue, we may send you an e-mail message to request more information about your feedback.
After the issues have been addressed, we may send you an email message to let you know that your feedback has been addressed.
Permanent link to this article: https://www.eurekalog.com/help/eurekalog/how_to_add_information_to_bug_report.php
|
|